Main concepts
These are main concepts of CodeBud that you should know
CodeBud / Package / Client
These terms will mean the CodeBud JS package itself and / or your application (client) that package is installed in.
GUI / Dashboard / Testing Panel / Website
These terms will mean CodeBud GUI for app inspection
API key / ApiKey
ApiKey is a unique string, which allows client to interact with the software infrastructure of CodeBud. The ApiKey is also used to identify the client.
Instruction
Instruction - an object that includes, among other things, a unique instruction ID and specific handler function that must be called on the client side in response to receiving the corresponding signal. You can use async function as handler function. CodeBud will await for such handlers execution to end, while execution progress will be displayed in GUI.
An instruction may have a "prototype". For example, the “login” prototype means that if there is a selected test account (in GUI), the account data will be automatically inserted into the event created from the instruction with such prototype.
The instruction can be default and special. Default instructions are the instructions that you define yourself. Special instructions are predefined.
Currently, there are 3 special instructions available:
- condition;
- delay;
- forwardData - A statement that links two events by passing the result of the previous Event into the params of the next one;
Instruction parameters
- Name
id
- Type
- string
- Description
Instruction ID is required and should be unique.
- Name
prototype
- Type
- [optional] "login" | "logout"
- Description
Instruction prototype.
- Name
parametersDescription
- Type
- [optional] object
- Description
This field is required if your handler function takes params.
Here you should describe your handler parameters object. List all the keys, and use built-in types for values. Available types: "number", "string", "object", "array", "boolean", and "?number", "?string",.. etc for optional fields.
- Name
description
- Type
- [optional] string
- Description
Use this for your convenience.
Add useful hints about corresponding instruction.
- Name
handler
- Type
- (data?: any) => any
- Description
Handler function that will be called every time when Event containing corresponding instruction id comes.
Should take 0 or 1 arg. Arg must be object that is defined with parametersDescription You can make handler async if you need to.
Example of instruction object
const instruction: Instruction = {
id: "helloWorldInstruction",
prototype: "login",
parametersDescription: {
year: "number" // Available types: "number", "string", "object", "array", "boolean", and "?number", "?string",.. etc for optional fields
},
description: "Some useful description text",
handler: (data: {year: number}) => {
console.log(`Hello, world ${data.year}`);
}
}
Instruction groups
Instruction can be organized into groups
function wait(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
const INSTRUCTIONS = [
{
id: "helloWorld",
handler: () => {
console.log("Hello, world!");
},
},
{
groupId: "AuthInstructions",
groupDescription: "Basic auth-related instructions",
groupColor: "#77a3b7",
groupInstructions: [
{
id: "login",
prototype: "login",
description: 'User login example instruction',
handler: () => {
console.log("Login event...");
}
},
{
id: "logout",
prototype: "logout",
description: "Logout user example instruction",
handler: () => {
console.log("Logout event...");
}
},
{ // Example of instruction with async handler function
id: "asyncTest",
description: "Async function that takes 100ms to perform console.log",
handler: async () => {
await wait(100);
return "Hey there!";
}
},
]
}
];
Event
Event - an object that includes, among other things, a unique event ID, id of the instruction being passed, and args (params) for the instruction handler. You are creating events by working with event / scenario constructor in GUI.
Scenario
Scenario (or sequence of events) - an object that includes a unique ID of the scenario, and a sequence of related events for execution. You are creating scenarios by working with scenario constructor in GUI. Sequence of events is automatically presented as scenario if it contains more than 1 event. Prepared scenarios can be saved into scenario collection or be downloaded as JSON file for easier use next time. Note: in order to use scenario collection, you should provide projectId.
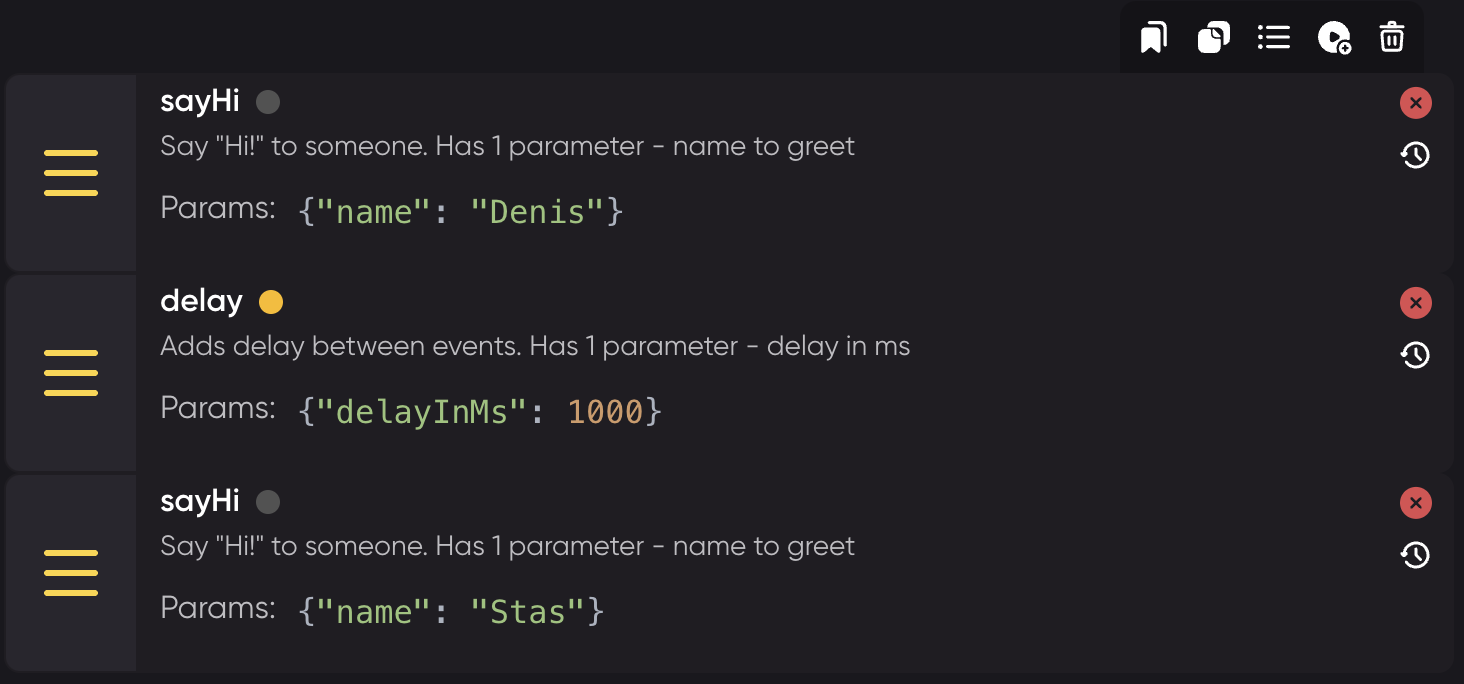
"Scenario collection" button is located in constructor menu (above the scenario constructor)
EventLog
EventLog is the event results report. It contains executed event data, ok flag, result (if there is one), error (if event execution failed) and timestamps of execution.
ScenarioLog
ScenarioLog is the scenario results report. It contains executed scenario data, ok flag, error (if scenario execution failed) and timestamps of execution.
You can see logs at the bottom of "Main" tab in the logger.
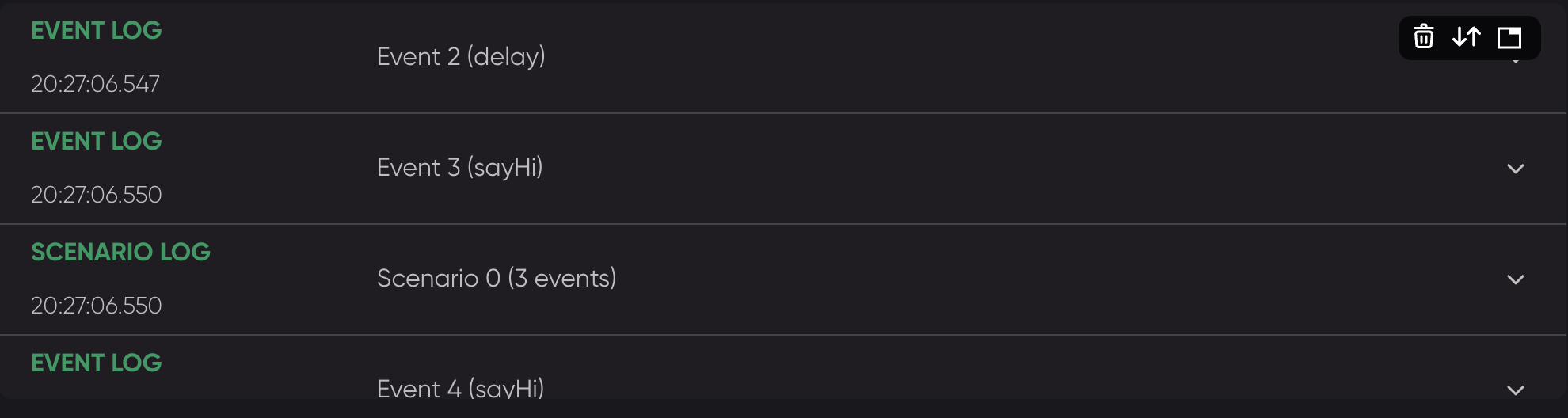
The logger
Projects
CodeBud projects allow you to work as a team and also open access to additional features:
- Scenario collections
- Feature management
How to create a project
First, on Control tab, create a new project and copy project id.
Second, pass project id to CodeBud.init method, like so:
CodeBud.init("YOUR API KEY HERE", INSTRUCTIONS, {
projectInfo: {
projectId: "YOUR PROJECT ID HERE"
},
remoteSettingsAutoUpdateInterval: 60000 // optional, sets the interval of auto-updating remote settings and personal projects settings
});
Now it's time to invite your team to the project. It can be done on Control tab. Note: don't forget to share projectId with your team.
Project ID purpose is to determine single exact project, while API Key purpose is to determine single running client and the CodeBud user account that key belongs to.
Development and production features
CodeBud provides many tools for app testing, inspection and debugging. It is important to remember, that most of those tools are designed to work in development mode only. Tools that are also available in production mode are marked with [prod] tag.
When to use production mode?
For example, when you use CodeBud in React project, and you are making production build, but you still need to use CodeBud's remote settings, so you cant fully disable CodeBud.
How to tell CodeBud that application is running in production mode:
In production mode CodeBud does not initialize socket connection with GUI panel, therefore prod mode is much more efficient.
CodeBud.init("YOUR API KEY HERE", INSTRUCTIONS, {
mode: "prod" // should be "dev" or "prod"
});